Contents
Hello there and welcome to this blog, today we will show you how to send emails using mailtrap in Symfony 6. Most web app has a sending email feature and during the development, there is always email testing, and that’s the mailtrap comes in. This tutorial will show you how to create an email and set up the mailtrap on your laravel app. Let’s have a few discussions.
What is Symfony? Symfony is a PHP framework used to develop web applications, APIs, microservices, and web services. Symfony is one of the leading PHP frameworks for creating websites and web applications.
Step 1: Install Symfony 6
First, select a folder in which you want Symfony to be installed then execute this command on Terminal or CMD to install:
Install via composer:
composer create-project symfony/website-skeleton symfony-6-mailtrap
Install via Symfony CLI:
symfony new symfony-6-mailtrap --full
Step 2: Install Package
After installing, we will then install the symfony/mailer package. This will be used in sending emails in our application.
composer require symfony/mailer
Step 3: Set Database ENV
Open the .env file and set the database configuration. We will be using MySQL in this tutorial. Uncomment the DATABASE_URL variable for MySQL and updates its configs. Make sure you comment out the other DATABASE_URL variables.
.env
DATABASE_URL="mysql://root:@127.0.0.1:3306/symfony_6_mailtrap?serverVersion=8&charset=utf8mb4"
Step 4: Set Mailer ENV
Before we can set the mailer env value, we must register an account in mailtrap and copy the username and password: Open the .env file and set the mailer configuration.
.env
MAILER_DSN=smtp://username:password@smtp.mailtrap.io:2525/?encryption=ssl&auth_mode=login
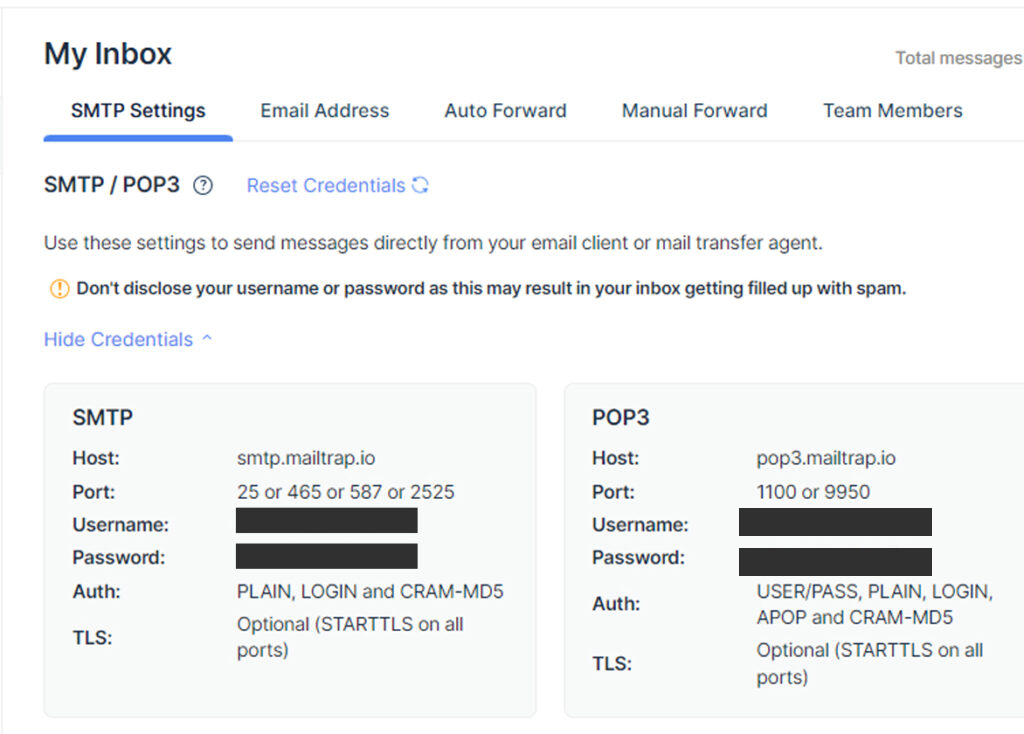
Step 5: Create A Controller
A Controller is the one responsible for receiving Request and returning Response. We will now create our controller, run this command to create a controller:
php bin/console make:controller MailerController
After creating a controller add these lines of codes:
<?php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Mailer\MailerInterface;
use Symfony\Component\Mime\Email;
use Symfony\Component\Routing\Annotation\Route;;
class MailerController extends AbstractController
{
#[Route('/send-email', name: 'app_send_email')]
public function index(MailerInterface $mailer): Response
{
$email = (new Email())
->from('sample-sender@binaryboxtuts.com')
->to('sample-recipient@binaryboxtuts.com')
->subject('Email Test')
->text('A sample email using mailtrap.');
$mailer->send($email);
return new Response(
'Email sent successfully'
);
}
}
Step 6: Run the Application
Now that we have completed the steps above we will now run the app. To run the app, execute this command:
symfony server:start
After successfully running your app, run this command to run the worker of messenger. The Messenger component helps applications send and receive messages to/from other applications or via message queues. You can read more about the symfony/messenger at this link.
php bin/console messenger:consume async
And finally, open this URL in your browser to test sending emails:
https://127.0.0.1:8000/send-email