Contents
Good day my fellow dev, today we will show you how to send emails using mailtrap in Laravel 8. Most web app has a sending email feature and during the development, there is always email testing, and that’s where the mailtrap comes in. This tutorial will show you how to create an email and set up mailtrap on your laravel app. Let’s have a few discussions.
Laravel is a free, open-source PHP Web Framework intended to develop web applications following the MVC (Model-View-Controller) architectural pattern. It is designed to make developing web apps faster and easier by using built-in features.
Mailtrap is used as a dummy SMTP server, it catches all the test emails that are being sent and can be viewed on its virtual inboxes. With mailtrap, email testing is safer and more manageable and avoids spamming real customers.
Step 1: Install Laravel 8
Run this command on Terminal or CMD to install Laravel via composer:
composer create-project laravel/laravel:^8.0 laravel-8-mailtrap
or via Laravel Installer:
laravel new laravel-8-mailtrap
Step 2: Update .env File
Before we can update the .env file, we must have an account in mailtrap to have a username and password. Once you are done registering just copy the username and password and put it on the .env file.
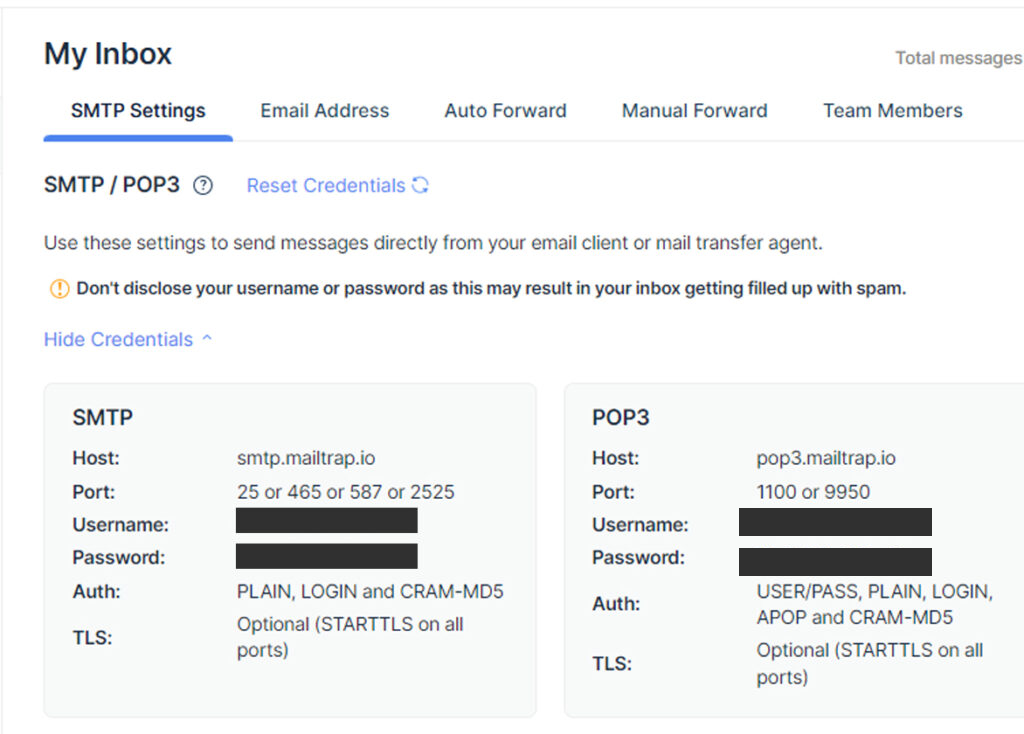
.env
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=*****************
MAIL_PASSWORD=*****************
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=info@binaryboxtus.com
MAIL_FROM_NAME="${APP_NAME}"
Step 3: Create A Markdown Mailable
Markdown mailable has laravel’s pre-built email UI components and has a markdown syntax. We will now generate a mailable class with a markdown template:
php artisan make:mail SampleBinaryboxtusEmail --markdown=emails.sample-binaryboxtus-email
Step 4: Register Route
We will not be creating a controller for this tutorial, we will directly test the sending email on the web routes. Open the file web.php and copy the codes below:
routes\web.php
<?php
use App\Mail\SampleBinaryboxtusEmail;
use Illuminate\Support\Facades\Route;
use Illuminate\Support\Facades\Mail;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get('/send-sample-email', function () {
Mail::to('tutorial@binaryboxtuts.com')->send(new SampleBinaryboxtusEmail());
});
Step 5: Run the Application
After finishing the steps above, you can now run your application by executing the code below:
php artisan serve
After successfully running your app, open this URL in your browser to test sending an email:
http://127.0.0.1:8000/send-sample-email