Contents
Introduction:
Good day, fellow dev, today I will be showing you how to make a Laravel 11 REST API. REST API is used for communication between client and server. REST stands for representational state transfer and API stands for application programming interface. REST API also known as RESTful API is a type of API that uses the REST architectural style, an API uses REST if it has these characteristics:
- Client-Server – The communication between Client and Server.
- Stateless – After the server completes an HTTP request, no session information is retained on the server.
- Cacheable – Response from the server can be cacheable or noncacheable.
- Uniform Interface – These are the constraints that simplify things. The constraints are Identification of the resources, resource manipulation through representations, self-descriptive messages, and hypermedia as the engine of the application state.
- Layered System – These are intermediaries between client and server like proxy servers, cache servers, etc.
- Code on Demand(Optional) – This is optional, the ability of the server to send executable codes to the client like Java applets or JavaScript code, etc.
Now you have a bit of insight about REST API. we will now proceed with creating this on Laravel 11.
Laravel is one of the most popular PHP frameworks and is widely used in web application development. It has been widely chosen because of its features and benefits. We won’t be talking about why we should Laravel but we will show you how to create a LARAVEL 11 REST API. This tutorial has been made for beginners or new to Laravel. Let’s begin!
Prerequisite:
- Composer
- MySQL
- PHP >= 8.2
Step 1: Install Laravel 11 using Composer
Run this command on Terminal or CMD to install Laravel via composer:
composer create-project laravel/laravel laravel-11-rest-api
or via Laravel Installer:
laravel new laravel-11-rest-api
Step 2: Setup Database Configuration
Inside the project root folder open the file .env and put the configuration for the database.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your database name(laravel_11_rest_api)
DB_USERNAME=your database username(root)
DB_PASSWORD=your database password(root)
Step 3: Create a Model with Migration
A model is a class that represents a table on a database.
Migration is like a version of your database.
Run this command on Terminal or CMD:
php artisan make:model Project --migration
After running this command you will find a file in this path “database/migrations” and update the code in that file.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('projects', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('projects');
}
};
Run the migration by executing the migrate
Artisan command:
php artisan migrate
Step 4: Enable API and Create an API Resource Controller
By default, laravel 11 API route is not enabled in laravel 11. We will enable the API:
php artisan install:api
After we enable the API, we will now create our controller. The controller will be responsible for handling HTTP incoming requests.
Run this command to create an API Resource Controller:
php artisan make:controller ProjectController --api
This command will generate a controller at “app/Http/Controllers/ProjectController.php”. It contains methods for each of the available resource operations. Open the file and insert these codes:
app/Http/Controllers/ProjectController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Project;
class ProjectController extends Controller
{
/**
* Display a listing of the resource.
*/
public function index()
{
$projects = Project::get();
return response()->json($projects);
}
/**
* Store a newly created resource in storage.
*/
public function store(Request $request)
{
$project = new Project();
$project->name = $request->name;
$project->description = $request->description;
$project->save();
return response()->json($project);
}
/**
* Display the specified resource.
*/
public function show(string $id)
{
$project = Project::find($id);
return response()->json($project);
}
/**
* Update the specified resource in storage.
*/
public function update(Request $request, string $id)
{
$project = Project::find($id);
$project->name = $request->name;
$project->description = $request->description;
$project->save();
return response()->json($project);
}
/**
* Remove the specified resource from storage.
*/
public function destroy(string $id)
{
Project::destroy($id);
return response()->json(['message' => 'Deleted']);
}
}
Step 5: Add an API resource route
We will be using the route file routes/api.php since we are creating an API. The routes inside routes/api.php are stateless and use the API middleware group.
When creating an API resource route, you must use the apiResource method to exclude the route that represents create and edit html templates.
Now we register the API resource routes:
routes/api.php
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProjectController;
Route::get('/user', function (Request $request) {
return $request->user();
})->middleware('auth:sanctum');
Route::apiResource('projects', ProjectController::class);
Step 6: Run the Laravel App
Run this command to start the Laravel App:
php artisan serve
After successfully running your app, open this URL in your browser:
http://localhost:8000
Test the API:
We will be using Postman for testing our API, but you can use you preferred tool.
POST Request – This request will create a new resource.
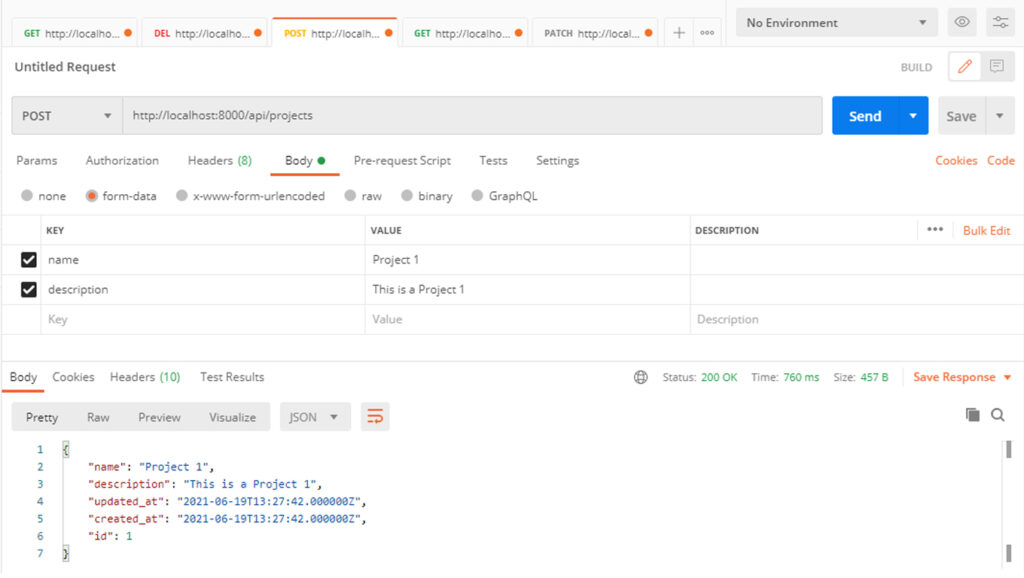
GET Request – This request will retrieve all the resources.
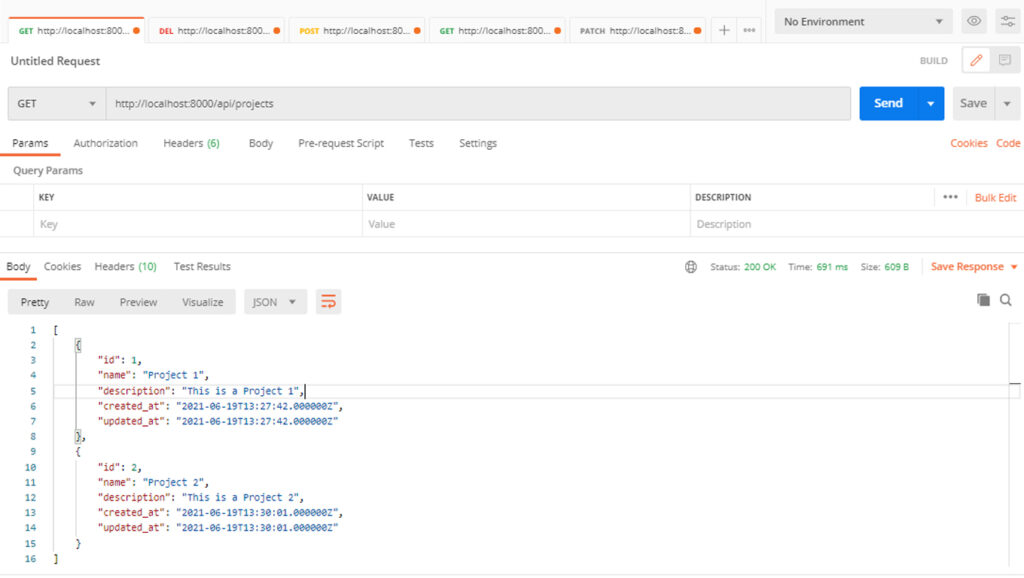
GET Request (with id) – This request will retrieve a particular resource.
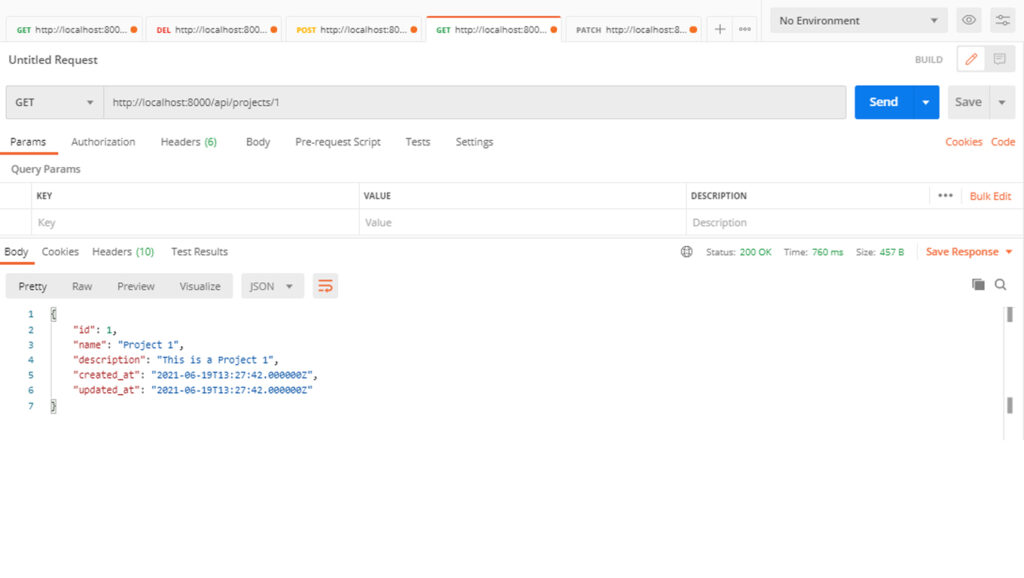
PATCH Request or you can use the PUT Request – This request will update a resource.
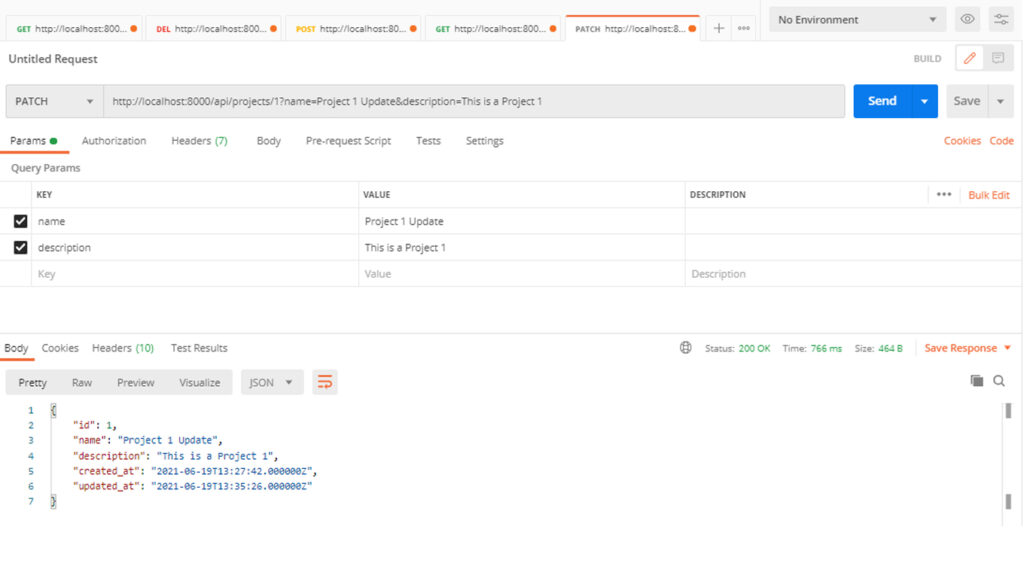
DELETE Request – This request will delete a resource.
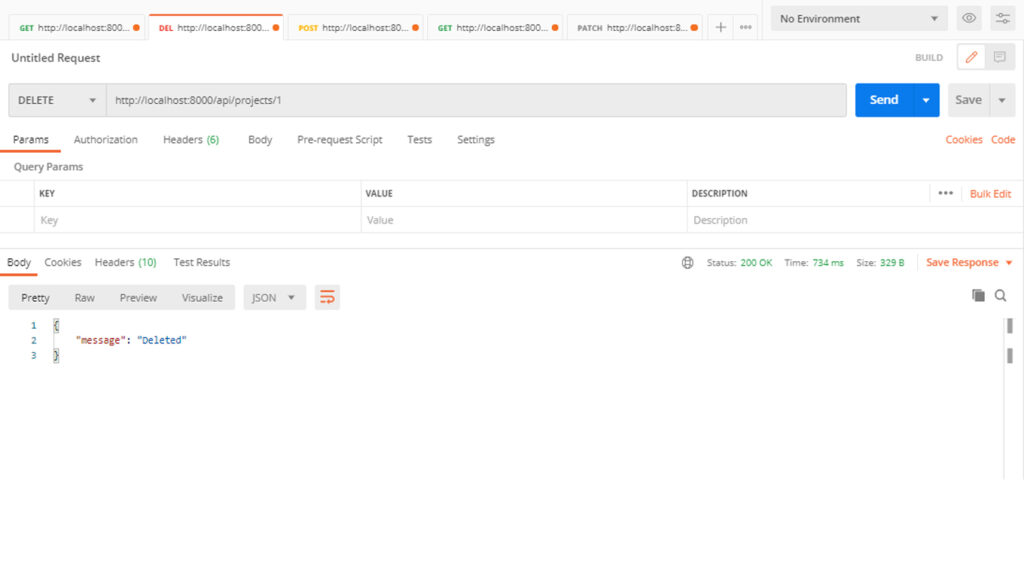