Contents
Good day fellow dev, in this blog I will be showing you how to generate PDF from HTML in Symfony 6. PDF has been one of the most commonly used document format today. Most of the web apps have a functionality that generates a PDF for the invoice. receipts, reports, and others.
What is PDF? It stands for Portable Document Format. It is a file format developed by Adobe to present or display documents in a manner independent of application software, hardware, and operating systems.
What is Symfony? Symfony is a PHP framework used to develop web applications, APIs, microservices, and web services. Symfony is one of the leading PHP frameworks for creating websites and web applications.
Prerequisite:
- Composer
- Symfony CLI
- PHP >= 8.0.2
Step 1: Install Symfony 6
First, select a folder that you want Symfony to be installed then execute this command on Terminal or CMD to install:
Install via composer:
composer create-project symfony/website-skeleton symfony-6-pdf
Install via Symfony CLI:
symfony new symfony-6-pdf--full
Step 2: Set Database Configuration
We must configure our database to avoid errors. Open the .env file and set database configuration. We will be using MySQL in this tutorial. Uncomment the DATABASE_URL variable for MySQL and updates its configs. Make sure you commented out the other DATABASE_URL variables.
.env
# In all environments, the following files are loaded if they exist,
# the latter taking precedence over the former:
#
# * .env contains default values for the environment variables needed by the app
# * .env.local uncommitted file with local overrides
# * .env.$APP_ENV committed environment-specific defaults
# * .env.$APP_ENV.local uncommitted environment-specific overrides
#
# Real environment variables win over .env files.
#
# DO NOT DEFINE PRODUCTION SECRETS IN THIS FILE NOR IN ANY OTHER COMMITTED FILES.
#
# Run "composer dump-env prod" to compile .env files for production use (requires symfony/flex >=1.2).
# https://symfony.com/doc/current/best_practices.html#use-environment-variables-for-infrastructure-configuration
###> symfony/framework-bundle ###
APP_ENV=dev
APP_SECRET=e0710317861221371d185cc932acd15b
###< symfony/framework-bundle ###
###> doctrine/doctrine-bundle ###
# Format described at https://www.doctrine-project.org/projects/doctrine-dbal/en/latest/reference/configuration.html#connecting-using-a-url
# IMPORTANT: You MUST configure your server version, either here or in config/packages/doctrine.yaml
#
# DATABASE_URL="sqlite:///%kernel.project_dir%/var/data.db"
# DATABASE_URL="mysql://db_user:db_password@127.0.0.1:3306/db_name?serverVersion=5.7"
DATABASE_URL="postgresql://db_user:db_password@127.0.0.1:5432/db_name?serverVersion=13&charset=utf8"
###< doctrine/doctrine-bundle ###
Step 3: Install DOMPDF Package
We will then install this package dompdf/dompdf, this package will be used in converting HTML into PDF. Run this comman to install the package:
composer require dompdf/dompdf
Step 4: Create A Controller
We will now create our controller, run this command to create a controller:
php bin/console make:controller PdfGenerator
After creating a controller add this codes:
<?php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Annotation\Route;
use Dompdf\Dompdf;
class PdfGeneratorController extends AbstractController
{
#[Route('/pdf/generator', name: 'app_pdf_generator')]
public function index(): Response
{
// return $this->render('pdf_generator/index.html.twig', [
// 'controller_name' => 'PdfGeneratorController',
// ]);
$data = [
'imageSrc' => $this->imageToBase64($this->getParameter('kernel.project_dir') . '/public/img/profile.png'),
'name' => 'John Doe',
'address' => 'USA',
'mobileNumber' => '000000000',
'email' => 'john.doe@email.com'
];
$html = $this->renderView('pdf_generator/index.html.twig', $data);
$dompdf = new Dompdf();
$dompdf->loadHtml($html);
$dompdf->render();
return new Response (
$dompdf->stream('resume', ["Attachment" => false]),
Response::HTTP_OK,
['Content-Type' => 'application/pdf']
);
}
private function imageToBase64($path) {
$path = $path;
$type = pathinfo($path, PATHINFO_EXTENSION);
$data = file_get_contents($path);
$base64 = 'data:image/' . $type . ';base64,' . base64_encode($data);
return $base64;
}
}
Step 5: Update The View
We will now update the view file that was created when we create a controller. Open the file /templates/pdf_generator/index.html.twig and remove the existing codes and change it into these:
/templates/pdf_generator/index.html.twig
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Resume</title>
</head>
<body>
<div style="margin: 0 auto;display: block;width: 500px;">
<table width="100%" border="1">
<tr>
<td colspan="2">
<img src="{{imageSrc}}" style="width:200px;">
</td>
</tr>
<tr>
<td>Name:</td>
<td>{{name}}</td>
</tr>
<tr>
<td>Address:</td>
<td>{{address}}</td>
</tr>
<tr>
<td>Mobile Number:</td>
<td>{{mobileNumber}}</td>
</tr>
<tr>
<td>Email:</td>
<td>{{email}}</td>
</tr>
</table>
</div>
</body>
</html>
Before proceeding to the next step, make sure you have an image file in the /public directory since we will be adding an image when we generate the PDF file. Here is my sample image used that is saved on /public/img/profile.png :
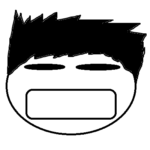
Step 6 : Run the Application
After finishing the steps above, you can now run your application by executing the command below:
symfony server:start
After successfully running your app, open this URL in your browser:
https://localhost:8000/pdf/generator
Screenshots:
Final Output
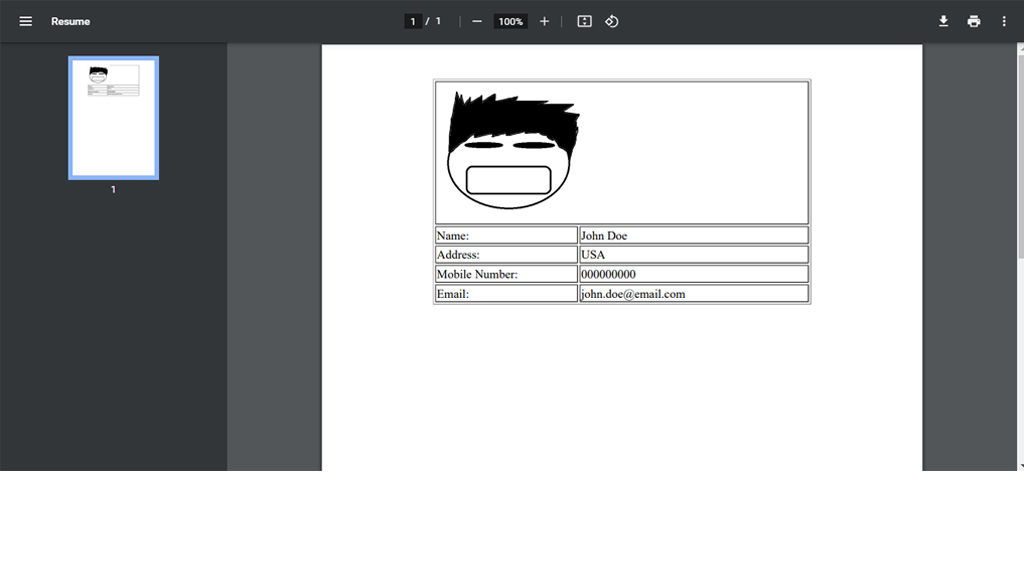