Contents
Introduction:
In this blog, You will learn how to develop a CodeIgniter 3 Simple AJAX CRUD App by following an easy step-by-step tutorial. But before that let us have an introduction:
CodeIgniter is a open-source web framework that is used for rapid web development. CodeIgniter follows the MVC (Model-View-Controller) architectural pattern. It is noted for its speed compared to other PHP web frameworks.
AJAX(Asynchronous JavaScript and XML) is a set of web development techniques that use many web technologies which allow web applications work in asynchronously.
CRUD is an acronym for CREATE, READ, UPDATE, DELETE. The four basic functions in any type of programming. CRUD typically refers to operations performed in a database.
- Create -Generate new record/s.
- Read – Reads or retrieve record/s.
- Update – Modify record/s.
- Delete – Destroy or remove record/s.
Before proceeding on this tutorial, you must have an environment already setup to run and install CodeIgniter 3. If you haven’t, please do read my blog –How to Install CodeIgniter 3 in XAMPP Easy Tutorial.
Step 1: Download CodeIgniter 3
First you must download the CodeIgniter 3 on this link.
After downloading, extract the file and rename the folder as “project-manager” or anything you prefer.
Step 2: Set Database Configuration
Before configuring the database, we must first create a database named project_manager and create a table named projects:
Run this SQL query to create the projects table:
CREATE TABLE `projects` (
`id` int(10) UNSIGNED NOT NULL,
`name` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL,
`description` text COLLATE utf8mb4_unicode_ci NOT NULL,
`created_at` timestamp NULL DEFAULT NULL,
`updated_at` timestamp NULL DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
Open the app folder and configure your database on this file “database.php“.
application/config/database.php
$db['default'] = array(
'dsn' => '',
'hostname' => 'localhost',
'username' => 'Your Username',
'password' => 'Your Password',
'database' => 'Your Database Name',
'dbdriver' => 'mysqli',
'dbprefix' => '',
'pconnect' => FALSE,
'db_debug' => (ENVIRONMENT !== 'production'),
'cache_on' => FALSE,
'cachedir' => '',
'char_set' => 'utf8',
'dbcollat' => 'utf8_general_ci',
'swap_pre' => '',
'encrypt' => FALSE,
'compress' => FALSE,
'stricton' => FALSE,
'failover' => array(),
'save_queries' => TRUE
);
Step 3: Update Config.php
Open the file config.php and find and update the base_url value.
application/config/config.php
$config['base_url'] = 'http://localhost/project-manager/index.php';
Step 4: Register Routes
And now we will register the routes for our CRUD app.
Open the routes.php file and register these route:
application/config/routes.php
$route['project'] = "project/index";
$route['project/create'] = "project/create";
$route['project/store']['post'] = "project/store";
$route['project/edit/(:num)'] = "project/edit/$1";
$route['project/update/(:num)']['put'] = "project/update/$1";
$route['project/delete/(:num)']['delete'] = "project/delete/$1";
Step 5: Create Controller
Go to this path “application/controllers/” and create a controller named Project.php. This will handle all our users actions. The controller will have these following methods.
- index()
- show_all()
- show($id)
- store()
- edit($id)
- update($id)
- delete($id)
After creating the controller, insert the codes below:
application/controllers/Project.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Project extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->library('form_validation');
$this->load->library('session');
$this->load->model('Project_model', 'project');
}
/*
View page of project
*/
public function index()
{
$data['title'] = "CodeIgniter Project Manager";
$this->load->view('projects',$data);
}
/*
Get all records
*/
public function show_all()
{
$projects = $this->project->get_all();
header('Content-Type: application/json');
echo json_encode($projects);
}
/*
Get a record
*/
public function show($id)
{
$project = $this->project->get($id);
header('Content-Type: application/json');
echo json_encode($project);
}
/*
Save the submitted record
*/
public function store()
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('description', 'Description', 'required');
if (!$this->form_validation->run())
{
http_response_code(412);
header('Content-Type: application/json');
echo json_encode([
'status' => 'error',
'errors' => validation_errors()
]);
} else {
$this->project->store();
header('Content-Type: application/json');
echo json_encode(['status' => "success"]);
}
}
/*
Edit a record
*/
public function edit($id)
{
$project = $this->project->get($id);
header('Content-Type: application/json');
echo json_encode($project);
}
/*
Update the submitted record
*/
public function update($id)
{
$this->form_validation->set_rules('name', 'Name', 'required');
$this->form_validation->set_rules('description', 'Description', 'required');
if (!$this->form_validation->run())
{
http_response_code(412);
header('Content-Type: application/json');
echo json_encode([
'status' => 'error',
'errors' => validation_errors()
]);
} else {
$this->project->update($id);
header('Content-Type: application/json');
echo json_encode(['status' => "success"]);
}
}
/*
Delete a record
*/
public function delete($id)
{
$item = $this->project->delete($id);
header('Content-Type: application/json');
echo json_encode(['status' => "success"]);
}
}
Step 6: Create Model
Go to this path “application/models/” and create a model named Project_model.php. This will handle all our query to the database. The model will have this following methods.
- get_all()
- store()
- get($id)
- update($id)
- delete($id)
After creating the model, insert the code below:
application/models/Project_model.php
<?php
class Project_model extends CI_Model{
public function __construct()
{
$this->load->database();
$this->load->helper('url');
}
/*
Get all the records from the database
*/
public function get_all()
{
$projects = $this->db->get("projects")->result();
return $projects;
}
/*
Store the record in the database
*/
public function store()
{
$data = [
'name' => $this->input->post('name'),
'description' => $this->input->post('description')
];
$result = $this->db->insert('projects', $data);
return $result;
}
/*
Get an specific record from the database
*/
public function get($id)
{
$project = $this->db->get_where('projects', ['id' => $id ])->row();
return $project;
}
/*
Update or Modify a record in the database
*/
public function update($id)
{
$data = [
'name' => $this->input->post('name'),
'description' => $this->input->post('description')
];
$result = $this->db->where('id',$id)->update('projects',$data);
return $result;
}
/*
Destroy or Remove a record in the database
*/
public function delete($id)
{
$result = $this->db->delete('projects', array('id' => $id));
return $result;
}
}
?>
Step 7: Create View Files
We will use Bootstrap to make our simple CRUD app presentable. Bootstrap is a free and most popular open-source CSS framework for building responsive and mobile-first web projects.
Create a file “projects.php” inside this following path “resources/views/”. Then insert the code below:
application/views/projects.php
<!DOCTYPE html>
<html lang="en">
<head>
<title><?php echo $title; ?></title>
<meta charset="utf-8">
<meta name="app-url" content="<?php echo base_url('/') ?>">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.4.1/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h2 class="text-center mt-5 mb-3"><?php echo $title; ?></h2>
<div class="card">
<div class="card-header">
<button class="btn btn-outline-primary" onclick="createProject()">
Create New Project
</button>
</div>
<div class="card-body">
<div id="alert-div">
</div>
<table class="table table-bordered">
<thead>
<tr>
<th>Name</th>
<th>Description</th>
<th width="240px">Action</th>
</tr>
</thead>
<tbody id="projects-table-body">
</tbody>
</table>
</div>
</div>
</div>
<!-- modal for creating and editing function -->
<div class="modal" tabindex="-1" role="dialog" id="form-modal">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Project Form</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div id="modal-alert-div">
</div>
<form>
<input type="hidden" name="update_id" id="update_id">
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name" name="name">
</div>
<div class="form-group">
<label for="description">Description</label>
<textarea class="form-control" id="description" rows="3" name="description"></textarea>
</div>
<button type="submit" class="btn btn-outline-primary" id="save-project-btn">Save Project</button>
</form>
</div>
</div>
</div>
</div>
<!-- view record modal -->
<div class="modal" tabindex="-1" role="dialog" id="view-modal">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Project Information</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<b>Name:</b>
<p id="name-info"></p>
<b>Description:</b>
<p id="description-info"></p>
</div>
</div>
</div>
</div>
<script type="text/javascript">
showAllProjects();
/*
This function will get all the project records
*/
function showAllProjects()
{
let url = $('meta[name=app-url]').attr("content") + "project/show-all";
$.ajax({
url: url,
type: "GET",
success: function(response) {
$("#projects-table-body").html("");
let projects = response;
for (var i = 0; i < projects.length; i++)
{
let showBtn = '<button ' +
' class="btn btn-outline-info" ' +
' onclick="showProject(' + projects[i].id + ')">Show' +
'</button> ';
let editBtn = '<button ' +
' class="btn btn-outline-success" ' +
' onclick="editProject(' + projects[i].id + ')">Edit' +
'</button> ';
let deleteBtn = '<button ' +
' class="btn btn-outline-danger" ' +
' onclick="destroyProject(' + projects[i].id + ')">Delete' +
'</button>';
let projectRow = '<tr>' +
'<td>' + projects[i].name + '</td>' +
'<td>' + projects[i].description + '</td>' +
'<td>' + showBtn + editBtn + deleteBtn + '</td>' +
'</tr>';
$("#projects-table-body").append(projectRow);
}
},
error: function(response) {
console.log(response)
}
});
}
/*
check if form submitted is for creating or updating
*/
$("#save-project-btn").click(function(event ){
event.preventDefault();
if($("#update_id").val() == null || $("#update_id").val() == "")
{
storeProject();
} else {
updateProject();
}
})
/*
show modal for creating a record and
empty the values of form and remove existing alerts
*/
function createProject()
{
$("#alert-div").html("");
$("#modal-alert-div").html("");
$("#update_id").val("");
$("#name").val("");
$("#description").val("");
$("#form-modal").modal('show');
}
/*
submit the form and will be stored to the database
*/
function storeProject()
{
$("#save-project-btn").prop('disabled', true);
let url = $('meta[name=app-url]').attr("content") + "/project/store";
let data = {
name: $("#name").val(),
description: $("#description").val(),
};
$.ajax({
url: url,
type: "POST",
data: data,
success: function(response) {
$("#save-project-btn").prop('disabled', false);
let successHtml = '<div class="alert alert-success" role="alert"><b>Project Created Successfully</b></div>';
$("#alert-div").html(successHtml);
$("#name").val("");
$("#description").val("");
showAllProjects();
$("#form-modal").modal('hide');
},
error: function(response) {
$("#save-project-btn").prop('disabled', false);
let responseData = JSON.parse(response.responseText);
console.log(responseData.errors);
if (typeof responseData.errors !== 'undefined')
{
let errorHtml = '<div class="alert alert-danger" role="alert">' +
'<b>Validation Error!</b>' +
responseData.errors +
'</div>';
$("#modal-alert-div").html(errorHtml);
}
}
});
}
/*
edit record function
it will get the existing value and show the project form
*/
function editProject(id)
{
let url = $('meta[name=app-url]').attr("content") + "project/edit/" + id ;
$.ajax({
url: url,
type: "GET",
success: function(response) {
let project = response;
$("#alert-div").html("");
$("#modal-alert-div").html("");
$("#update_id").val(project.id);
$("#name").val(project.name);
$("#description").val(project.description);
$("#form-modal").modal('show');
},
error: function(response) {
}
});
}
/*
sumbit the form and will update a record
*/
function updateProject()
{
$("#save-project-btn").prop('disabled', true);
let url = $('meta[name=app-url]').attr("content") + "project/update/" + $("#update_id").val();
let data = {
id: $("#update_id").val(),
name: $("#name").val(),
description: $("#description").val(),
};
$.ajax({
url: url,
type: "POST",
data: data,
success: function(response) {
$("#save-project-btn").prop('disabled', false);
let successHtml = '<div class="alert alert-success" role="alert"><b>Project Updated Successfully</b></div>';
$("#alert-div").html(successHtml);
$("#name").val("");
$("#description").val("");
showAllProjects();
$("#form-modal").modal('hide');
},
error: function(response) {
/*
show validation error
*/
$("#save-project-btn").prop('disabled', false);
let responseData = JSON.parse(response.responseText);
console.log(responseData.errors);
if (typeof responseData.errors !== 'undefined')
{
let errorHtml = '<div class="alert alert-danger" role="alert">' +
'<b>Validation Error!</b>' +
responseData.errors +
'</div>';
$("#modal-alert-div").html(errorHtml);
}
}
});
}
/*
get and display the record info on modal
*/
function showProject(id)
{
$("#name-info").html("");
$("#description-info").html("");
let url = $('meta[name=app-url]').attr("content") + "project/show/" + id +"";
$.ajax({
url: url,
type: "GET",
success: function(response) {
console.log(response);
let project = response;
$("#name-info").html(project.name);
$("#description-info").html(project.description);
$("#view-modal").modal('show');
},
error: function(response) {
console.log(response)
}
});
}
/*
delete record function
*/
function destroyProject(id)
{
let url = $('meta[name=app-url]').attr("content") + "/project/delete/" + id;
let data = {
name: $("#name").val(),
description: $("#description").val(),
};
$.ajax({
url: url,
type: "DELETE",
data: data,
success: function(response) {
let successHtml = '<div class="alert alert-success" role="alert"><b>Project Deleted Successfully</b></div>';
$("#alert-div").html(successHtml);
showAllProjects();
},
error: function(response) {
console.log(response)
}
});
}
</script>
</body>
</html>
Finally all the components are done. Now we can test our CodeIgniter 3 Simple CRUD App by browsing this URL:
http://localhost/project-manager-ajax/index.php/project
Screenshots:
CodeIgniter 3 Simple AJAX CRUD(index page):
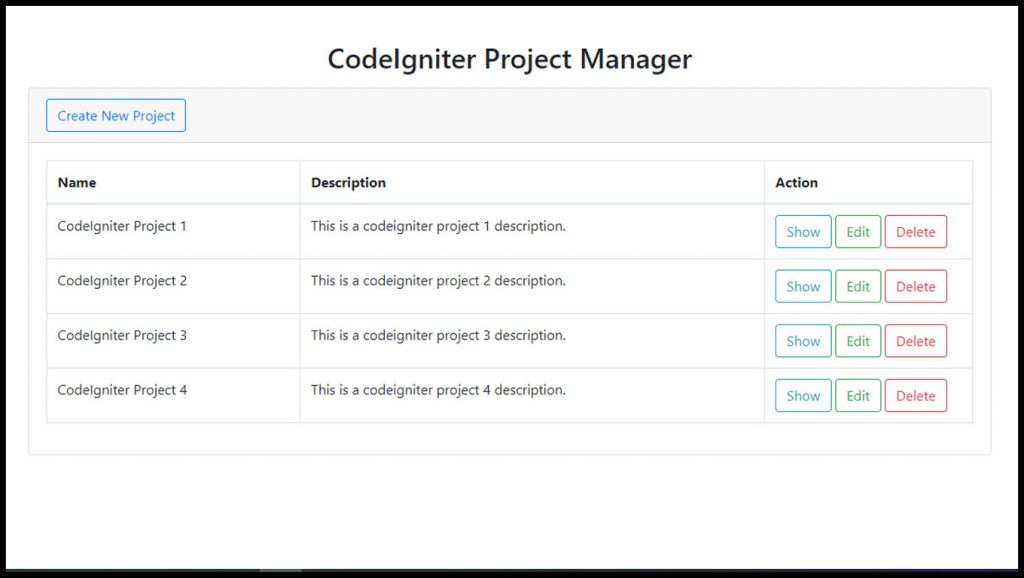
CodeIgniter 3 Simple AJAX CRUD (project create form modal):
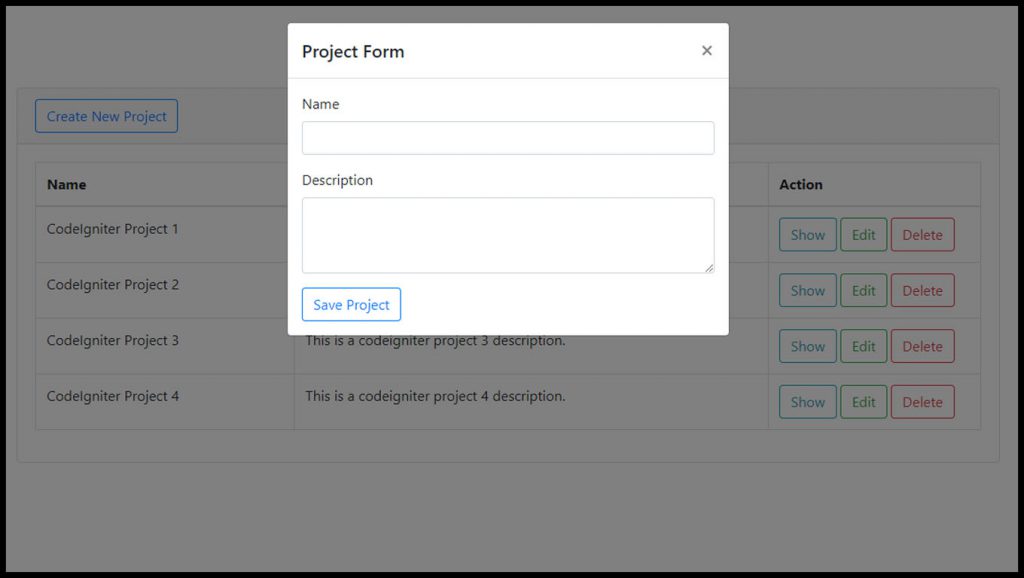
CodeIgniter 3 Simple AJAX CRUD(edit project form modal):
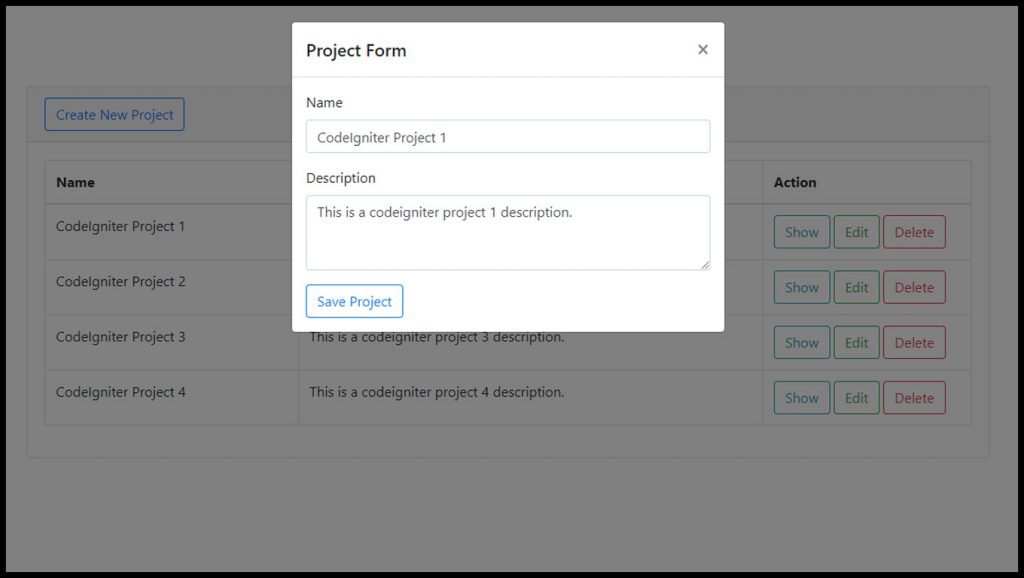
CodeIgniter 3 Simple AJAX CRUD (show project information modal):
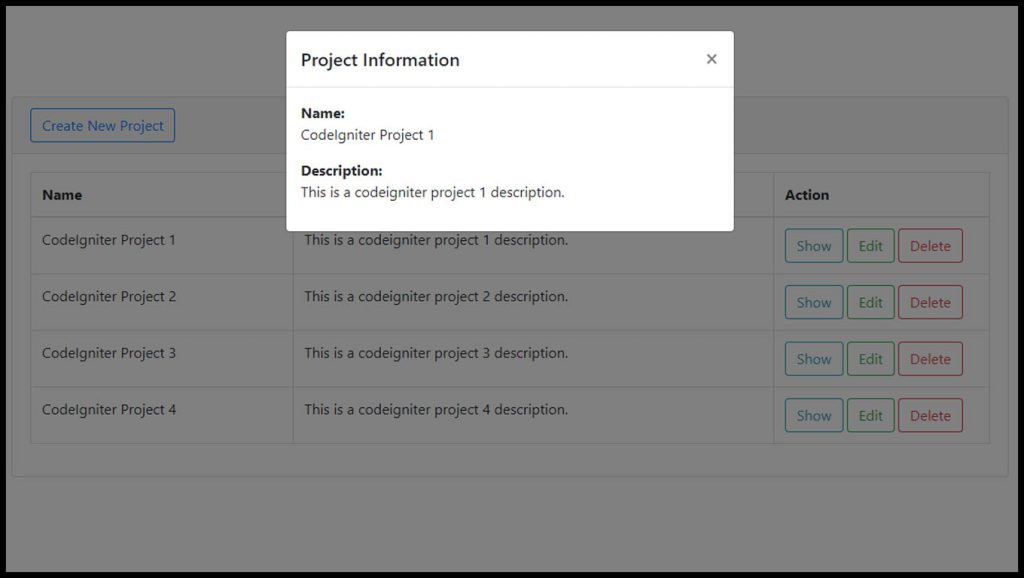