Contents
Good day, welcome to this blog. Today I will be showing you how to develop a single-page application using Nuxt. Before we proceed let’s have a little bit of discussion.
Nuxt is a framework for building Vue.js applications. It provides a higher-level abstraction for developing Vue applications with features that enhance the development process.
A Single Page Application (SPA) is a web application or website that utilizes only a single page and dynamically changes its content. The page does not reload unlike Multiple Page Application (MPA) which reloads pages to display new information.
Prerequisite:
- node >= v18.0.0
- npx
Step 1: Create A Nuxt Project
First, select a folder that you want the nuxt project to be created then execute this command on Terminal or CMD :
npx nuxi@latest init my-website
Step 2: Install packages
After creating a fresh nuxt project, go to the nuxt project folder and install these packages:
- bootstrap – a package of bootstrap framework
To install these packages. execute this command on Terminal or CMD.
npm i bootstrap
Update nuxt.config.ts:
// https://nuxt.com/docs/api/configuration/nuxt-config
export default defineNuxtConfig({
devtools: { enabled: true },
css: [
'bootstrap/dist/css/bootstrap.css',
],
})
Step 3: Create Layouts, Components And Pages
We will now create the files for our app, refer to the image below for the file structure.
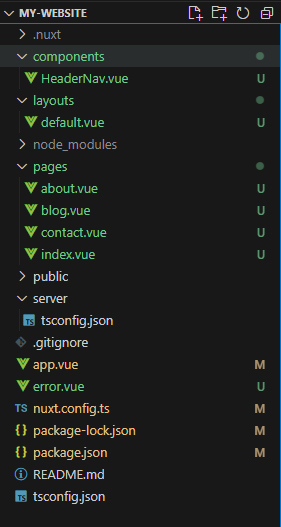
You can create your file structure but for this tutorial just follow along.
Let’s update the App.vue file:
src/App.vue
<template>
<NuxtLayout>
<Head>
<title>NUXT CRUD APP</title>
</Head>
<NuxtPage />
</NuxtLayout>
</template>
Create a directory named layouts and inside it create a file default.vue. This will be our main layout
layouts/default.vue
<template>
<div class="container">
<slot/>
</div>
</template>
Create a directory named components and inside it create a file HeaderNav.vue.
components/HeaderNav.vue
<script setup lang="ts">
const pageLinks = [
{
"name": "Home",
"url" :"/",
},
{
"name": "Blog",
"url" :"/blog",
},
{
"name": "About",
"url" :"/about",
},
{
"name": "Contact",
"url" :"/contact",
},
]
</script>
<template>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<router-link to="/" class="navbar-brand">Binaryboxtuts</router-link>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul v-for="(pageLink, index) in pageLinks" :key="index" class="navbar-nav mr-auto">
<li class="nav-item">
<router-link
:to="pageLink.url"
class="nav-link"
:class="$route.path === pageLink.url ? 'active' : ''">
{{pageLink.name}}
</router-link>
</li>
</ul>
</div>
</nav>
</template>
Create directory pages. Inside the folder let’s create these files for our pages:
- index.vue
- blog.vue
- about.vue
- contact.vue
And for our 404 page we will create a file on our root directory, name the file error.vue
error.vue
<script setup lang="ts">
import type { NuxtError } from '#app'
const props = defineProps({
error: Object as () => NuxtError
})
const handleError = () => clearError({ redirect: '/' })
</script>
<template>
<NuxtLayout>
<Head>
<title>PAGE ERROR</title>
</Head>
<header-nav/>
<div class="container" align="center">
<h2 v-if="error.statusCode == 404" class="text-center mt-5 mb-3" >404 | Page Not Found</h2>
<h2 v-else class="text-center mt-5 mb-3" >Something went wrong</h2>
<button @click="handleError" class="btn btn-outline-primary">Go to home</button>
</div>
</NuxtLayout>
</template>
pages/index.vue
<script setup lang="ts">
//
</script>
<template>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">Home Page</h2>
</div>
</template>
pages/blog.vue
<script setup lang="ts">
//
</script>
<template>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">Blog Page</h2>
</div>
</template>
pages/about.vue
<script setup lang="ts">
//
</script>
<template>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">About Page</h2>
</div>
</template>
src/pages/contact.vue
<script setup lang="ts">
//
</script>
<template>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">Contact Page</h2>
</div>
</template>
Step 4: Run the app
We’re all done, what is left is to run the app.
npm run dev
Open this URL:
http://localhost:3000/
Screenshots:
Home Page
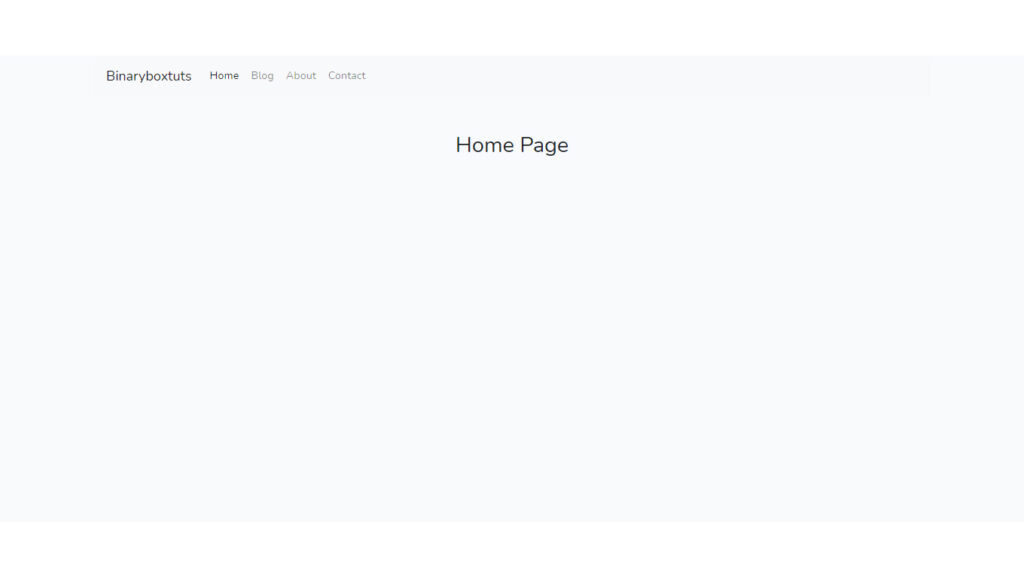
Blog Page
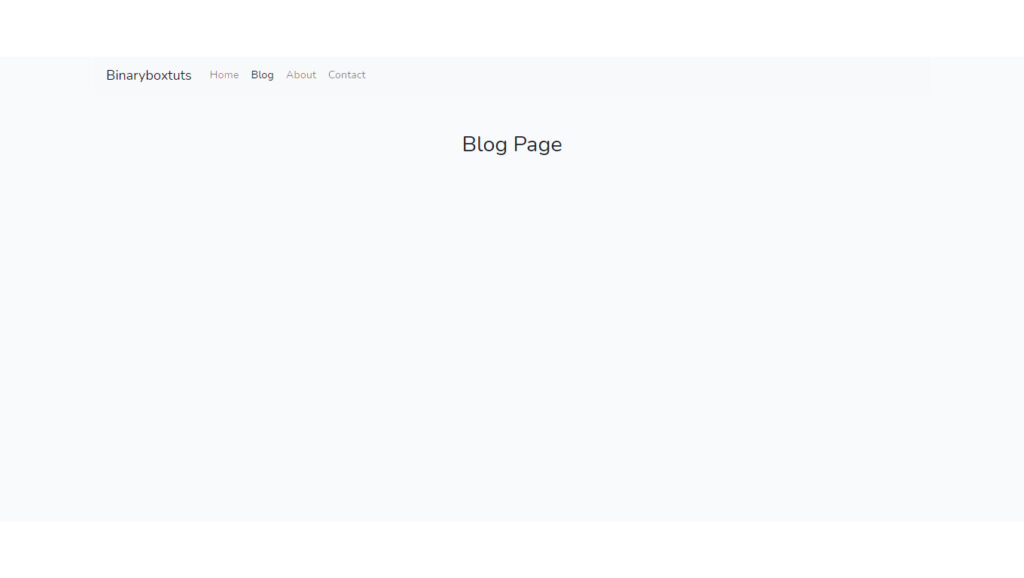
About Page
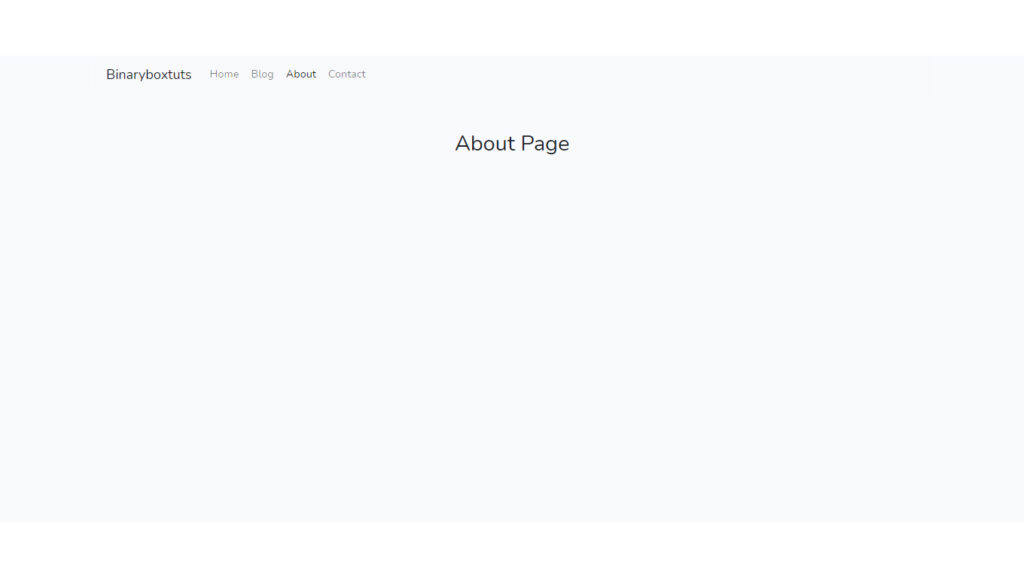
Contact Page
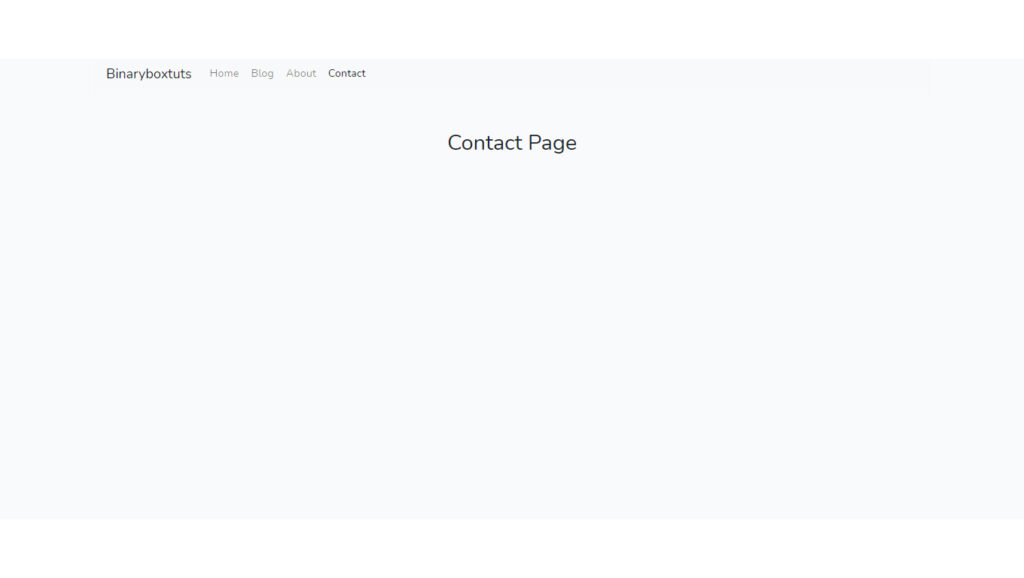
404 Page
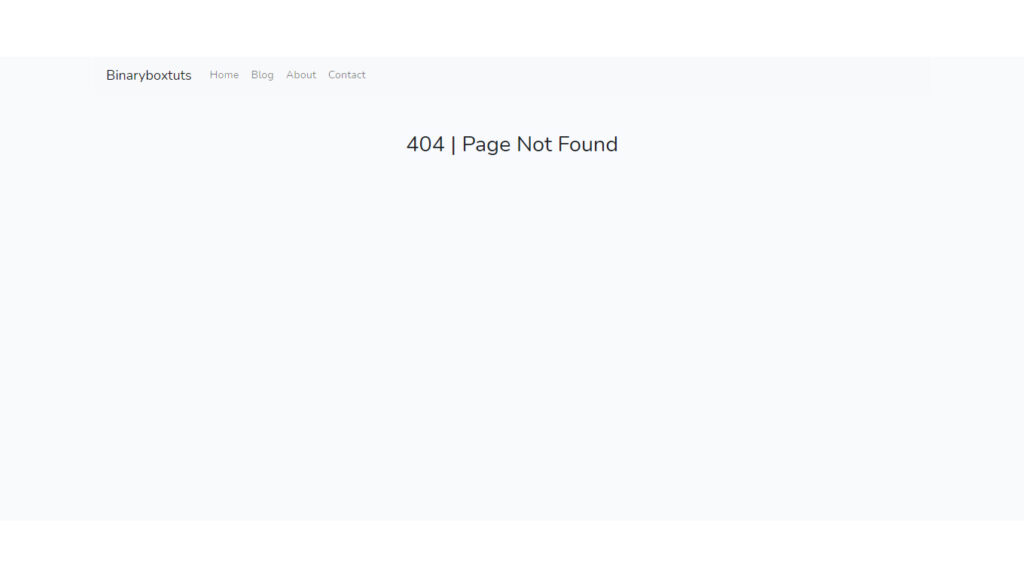