Contents
Good day, welcome to this blog. Today I will be showing you how to develop a single-page application using Vue.js. Before we proceed let’s have a little bit of discussion.
Vue JS or also called vue.js is an open-source JavaScript library used for building user interfaces(UI). It is one of the most popular JavaScript libraries for building the front end.
A Single Page Application (SPA) is a web application or website that utilizes only a single page and dynamically changes its content. The page does not reload unlike Multiple Page Application (MPA) which reloads pages to display new information.
Prerequisite:
- node >= v16.10.0
- @vue/cli
Step 1: Create A Vue.js Project
First, select a folder that you want the Vue.js project to be created then execute this command on Terminal or CMD :
vue create my-website
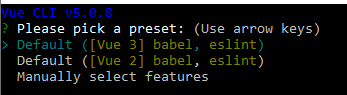
Step 2: Install packages
After creating a fresh vue.js project, go to the vue.js project folder and install these packages:
- vue-router – a package used for routing and the official router for vue.js
- bootstrap – a package of bootstrap framework
To install these packages. execute this command on Terminal or CMD.
npm i bootstrap
npm i vue-router
Step 3: Routing and Components
We will now create the component, refer to the image below for the file structure.
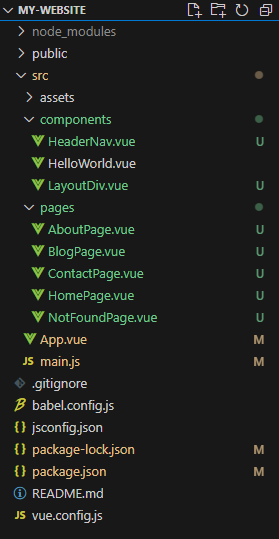
You can create your file structure but for this tutorial just follow along.
Let’s update the App.vue file:
src/App.vue
<template>
<div id="app">
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'App',
};
</script>
Let’s update the main.js file – We will add the routes here:
src/main.js
import { createApp } from 'vue';
import App from './App.vue';
import 'bootstrap/dist/css/bootstrap.css';
import { createRouter, createWebHistory } from 'vue-router';
import HomePage from './pages/HomePage';
import BlogPage from './pages/BlogPage';
import AboutPage from './pages/AboutPage';
import ContactPage from './pages/ContactPage';
import NotFoundPage from './pages/NotFoundPage';
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/', component: HomePage },
{ path: '/blog', component: BlogPage },
{ path: '/about', component: AboutPage },
{ path: '/contact', component: ContactPage },
{ path: '/:pathMatch(.*)*', component: NotFoundPage },
],
});
createApp(App).use(router).mount('#app');
Let’s create 2 files named LayoutDiv.vue and HeaderNav.vue inside the /src/components directory– this will serve as a template.
src/components/LayoutDiv.vue
<template>
<div class="container">
<slot></slot>
</div>
</template>
<script>
export default {
name: 'LayoutDiv',
};
</script>
src/components/HeaderNav.vue
<template>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<router-link to="/" class="navbar-brand">Binaryboxtuts</router-link>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul v-for="(pageLink, index) in pageLinks" :key="index" class="navbar-nav mr-auto">
<li class="nav-item">
<router-link
:to="pageLink.url"
class="nav-link"
:class="$route.path === pageLink.url ? 'active' : ''">
{{pageLink.name}}
</router-link>
</li>
</ul>
</div>
</nav>
</template>
<script>
export default {
name: 'HeaderNav',
data() {
return {
pageLinks :[
{
"name": "Home",
"url" :"/",
},
{
"name": "Blog",
"url" :"/blog",
},
{
"name": "About",
"url" :"/about",
},
{
"name": "Contact",
"url" :"/contact",
},
]
};
},
};
</script>
We will now create a directory src/pages. Inside the folder let’s create these files for our pages:
- HomePage.vue
- BlogPage.vue
- AboutPage.vue
- ContactPage.vue
- NotFoundPage.vue
src/pages/HomePage.vue
<template>
<layout-div>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">Home Page</h2>
</div>
</layout-div>
</template>
<script>
import LayoutDiv from './../components/LayoutDiv.vue';
import HeaderNav from '../components/HeaderNav.vue';
export default {
name: 'HomePage',
components: {
LayoutDiv,
HeaderNav,
},
}
</script>
src/pages/BlogPage.vue
<template>
<layout-div>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">Blog Page</h2>
</div>
</layout-div>
</template>
<script>
import LayoutDiv from '../components/LayoutDiv.vue';
import HeaderNav from '../components/HeaderNav.vue';
export default {
name: 'BlogPage',
components: {
LayoutDiv,
HeaderNav,
},
}
</script>
src/pages/AboutPage.vue
<template>
<layout-div>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">About Page</h2>
</div>
</layout-div>
</template>
<script>
import LayoutDiv from '../components/LayoutDiv.vue';
import HeaderNav from '../components/HeaderNav.vue';
export default {
name: 'AboutPage',
components: {
LayoutDiv,
HeaderNav,
},
}
</script>
src/pages/ContactPage.vue
<template>
<layout-div>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">Contact Page</h2>
</div>
</layout-div>
</template>
<script>
import LayoutDiv from '../components/LayoutDiv.vue';
import HeaderNav from '../components/HeaderNav.vue';
export default {
name: 'ContactPage',
components: {
LayoutDiv,
HeaderNav,
},
}
</script>
src/pages/NotFoundPage.vue
<template>
<layout-div>
<header-nav/>
<div class="container">
<h2 class="text-center mt-5 mb-3">404 | Page Not Found</h2>
</div>
</layout-div>
</template>
<script>
import LayoutDiv from '../components/LayoutDiv.vue';
import HeaderNav from '../components/HeaderNav.vue';
export default {
name: 'NotFoundPage',
components: {
LayoutDiv,
HeaderNav,
},
}
</script>
Step 4: Run the app
We’re all done, what is left is to run the app.
npm run serve
Open this URL:
http://localhost:8080/
Screenshots:
Home Page
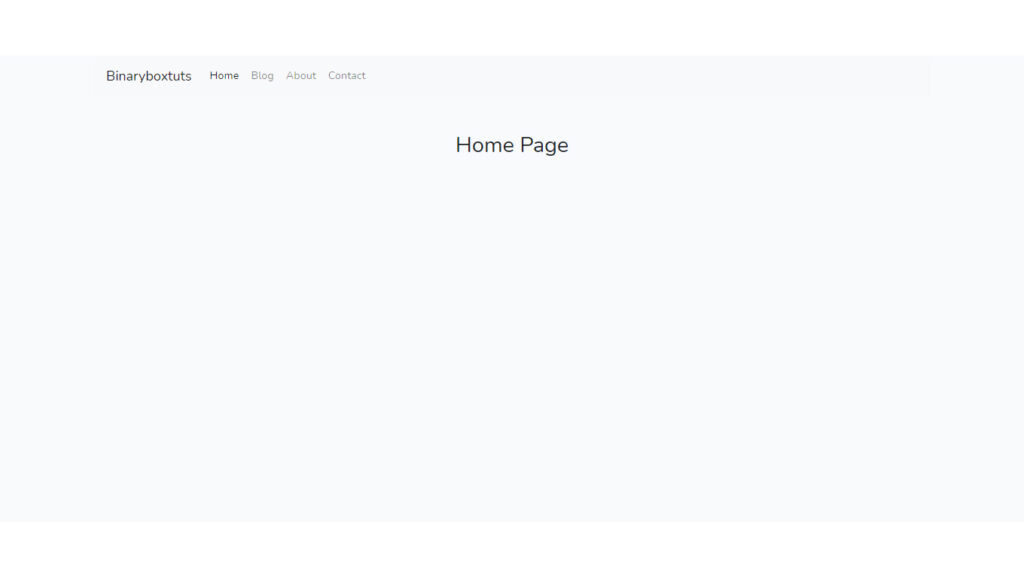
Blog Page
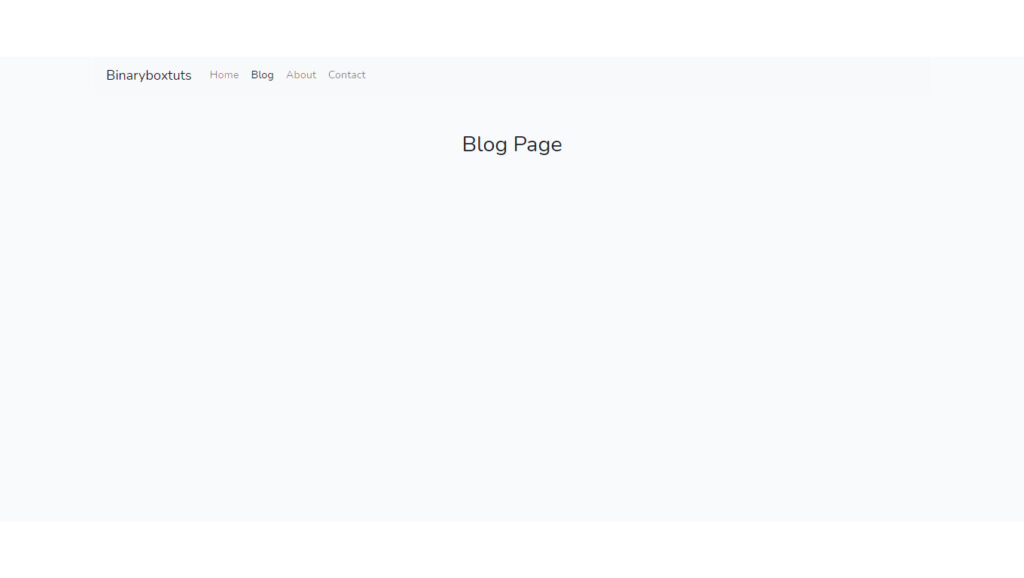
About Page
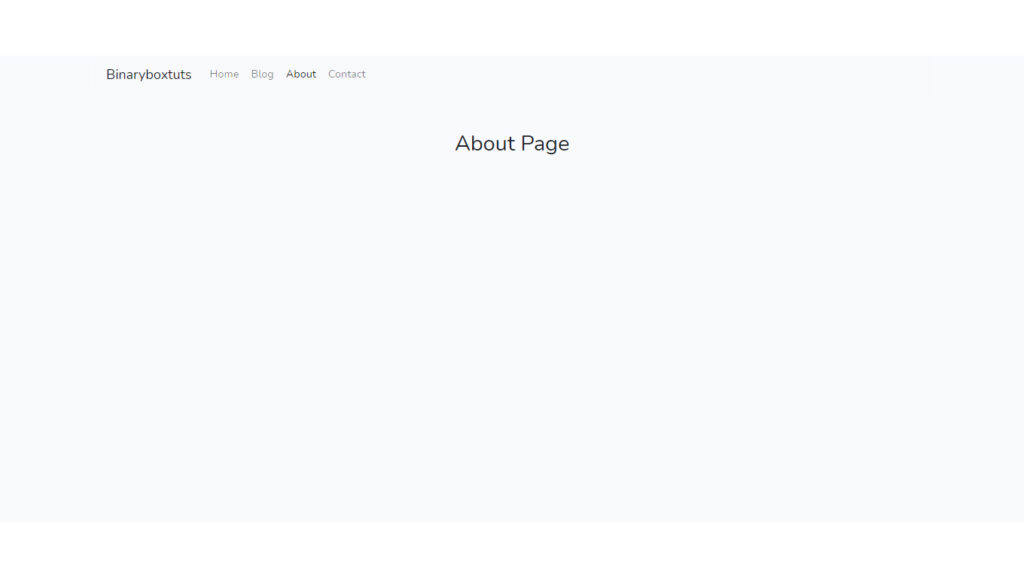
Contact Page
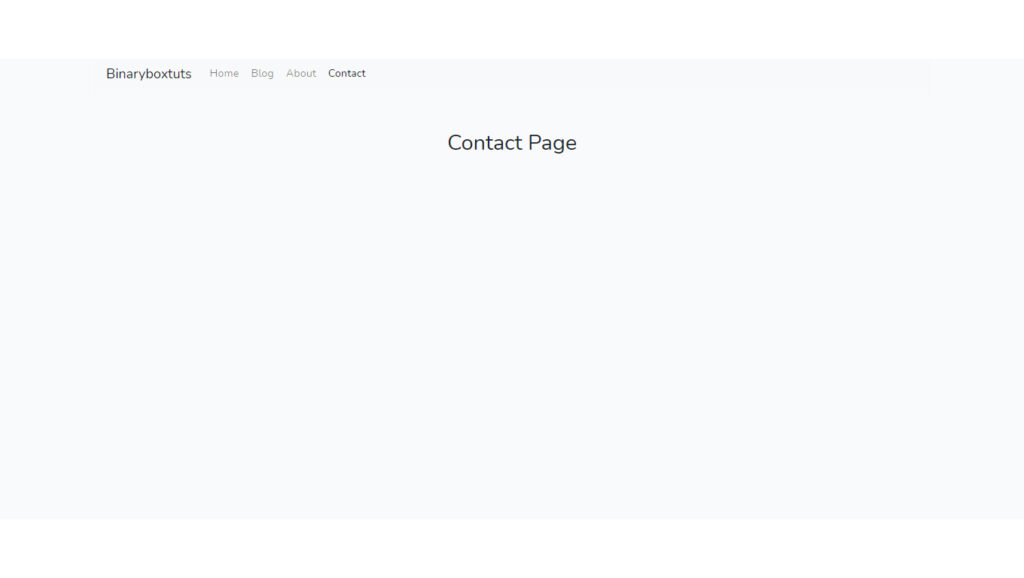
404 Page
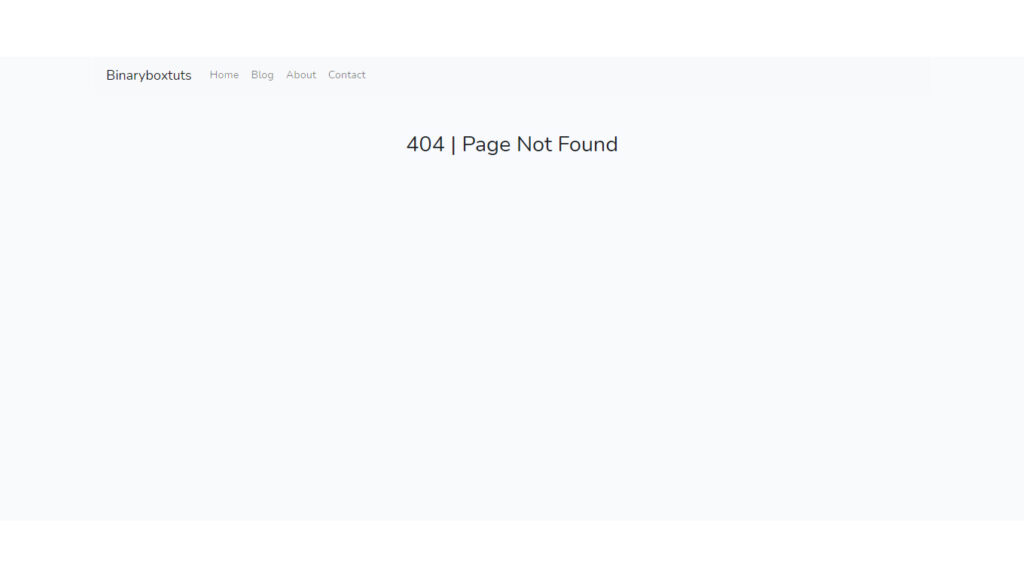